Mastering Lazy Loading in Angular: A Comprehensive Guide
Learn everything you need to know about Lazy Loading in Angular and take your skills to the next level with this in-depth guide.
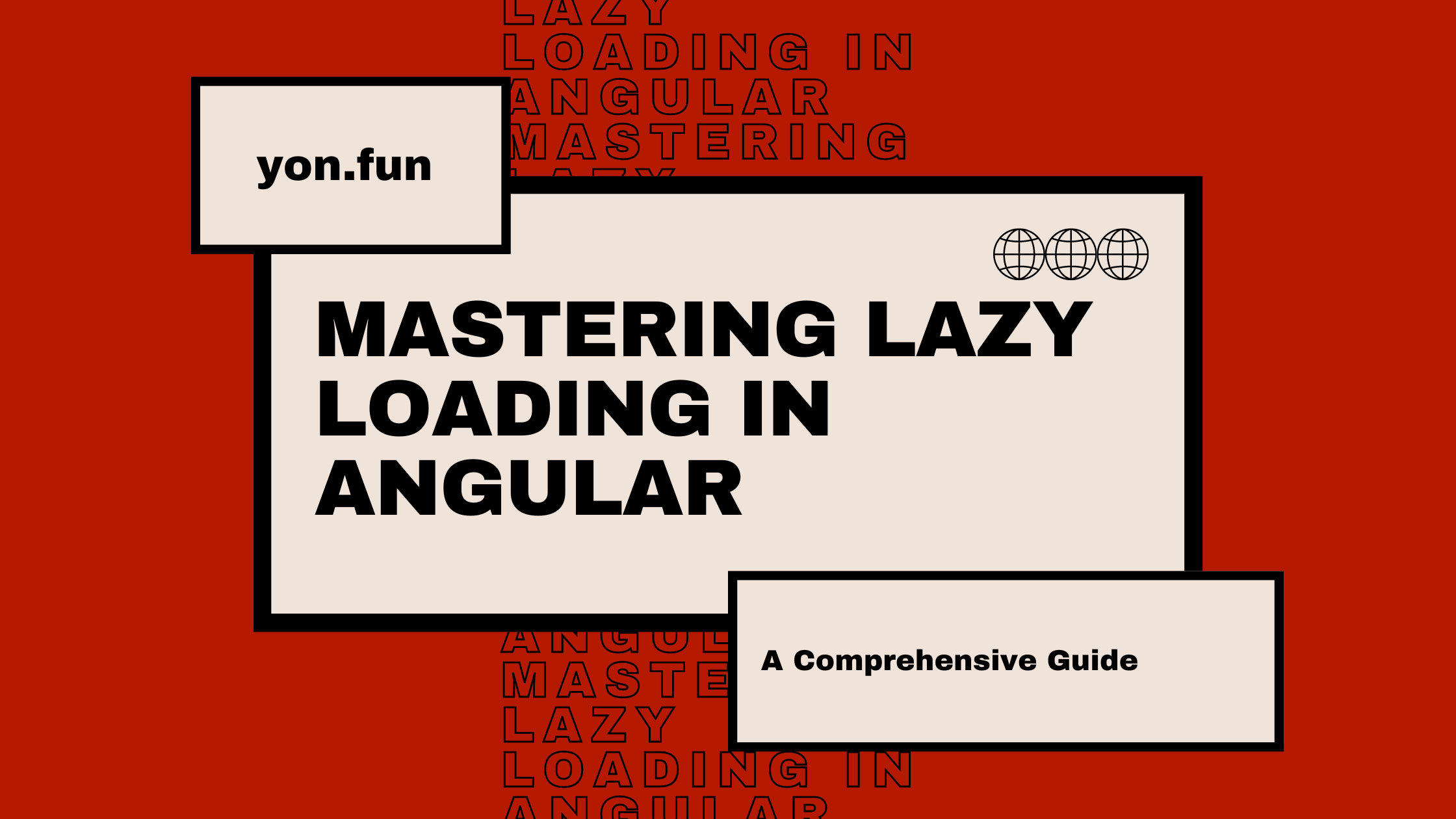
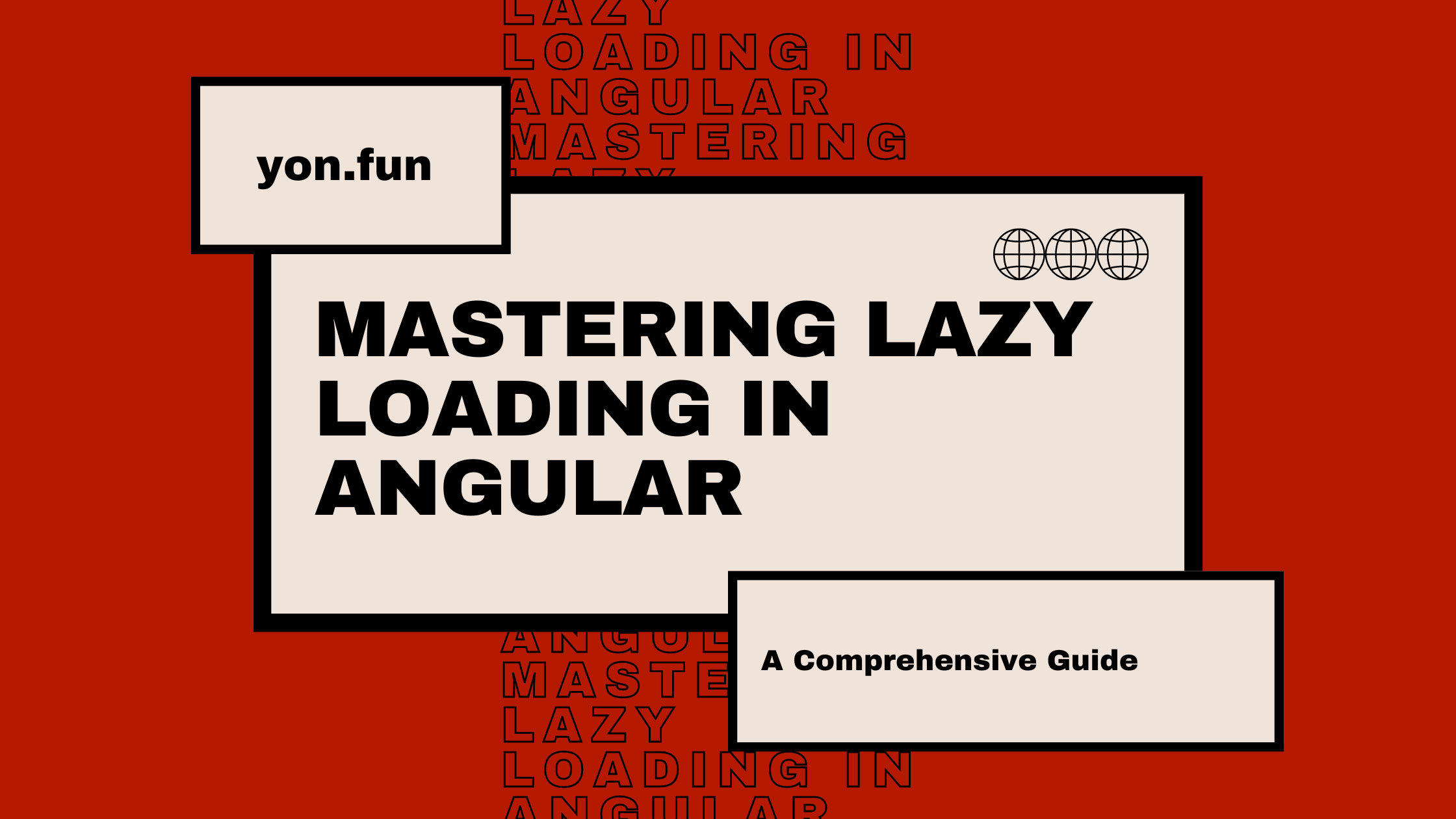
Lazy loading is a key performance optimization approach in Angular applications. This implies postponing the loading of feature modules until they are required.
This activity helps increase loads or page times and decreases memory usage, resulting in more user-friendly programs.
Familiarity with AOT, lazy loading, and its applications is essential for creating highly performant and scalable Angular applications.
Lazy Loading has the strong advantage of enhancing the first load times because only essential elements of the application are loaded. This is particularly helpful in applications that entail extensive use of features and the implementation of various functions.
Lazy loading is effective in achieving the goal of delivering faster UI interactions. Doing so minimizes the extent to which large swaths of code need to be offloaded at the initiation of the program’s execution.
Further, in this method, memory utilization is also reduced, and therefore, they increase the efficiency of the application.
Lazy loading does the following to an application:
- It makes the application faster.
- Takes less time to load.
- Uses less memory.
For instance, if the given application contains numerous components and routes, it would be unwise to load all the components at the same time since this would slow down the application.
Lazy loading helps in this by loading only the modules (and not only) that are required for the first instance, and this makes the application turn interactive much earlier.
This technique is particularly beneficial for applications that include large initial loading of data since it facilitates more efficient distribution of the resources.
Understanding Lazy Loading in Angular
Angular has introduced lazy loading through its routing mechanism and the modularity approach the Framework takes.
In Angular applications, the structure is developed regarding feature modules relative to a certain section or functionality. These modules can be lazy loaded with the help of RouterModule
and loadChildren
properties.
This means that the mentioned modules will only be loaded when the concerned route is being processed.
How Lazy Loading Works
The Lazy loading in angular is performed through routing.
With the help of routes and property loadChildren
the developers can declare which module should be applied when the user is at a particular route.
This keeps the initial loading bundle small and gets it on the screen faster as it is an important aspect of a website to enhance the user experience.
Setting Up Lazy Loading
Follow these steps to set up Lazy Loading:
Create a Feature Module
Usually, one creates a new feature module using the Angular CLI command when adding a new section, module, or feature to the application.
For instance, to make an ‘Admin’ module one has to sort the taking after the command:
ng generate module admin --route admin --module app.module
Configure Routes
Note that corresponding references to this book appear in the app-routing.module
. In the ‘ts’ file, set the routes to make the feature module lazy load using the loadChildren
parameter:
const routes: Routes = [
{
path: 'admin',
loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule)
}
];
Organize Module Files
Ensure that the feature module contains routing modules and the component files are arranged appropriately.
Go check the official documentation page for more in-depth lazy loading configuration.
Tips for Smooth Configuring of Lazy Loading
For optimal performance, consider the following tips:
Split Large Modules
Splitting the huge feature modules into the sub-feature modules leads to faster loads.
Loading large modules at once becomes quite cumbersome, particularly when a module incorporates several sub-modules, services, and dependencies.
By dividing these modules into smaller sub-modules, only the fragments necessary for the application’s functioning are loaded into the system at the time. This reduces the amount of data that is initially loaded at the time the application is launched for use, making it more interactive.
Use Route Guards
In Angular, the role of the route guards is to permit or deny access to particular routes in your application, depending on the user’s role and authenticity.
Applying route guards for the lazy-loaded modules adds an extra layer of security to the specific parts of the application. This is beneficial because it minimizes the loading of modules for a user that does not belong to the admin bracket. A code example of CanActivate Guardexport const yourGuardFunction: CanActivateFn = (
next: ActivatedRouteSnapshot,
state: RouterStateSnapshot) => {
// your logic goes here
}
// routers.modules.ts
{
path: '/your-path',
component: YourComponent,
canActivate: [yourGuardFunction],
}
Route guards are of six types, namely CanActivate
, CanActivateChild
, CanDeactivate
, CanMatch
, Resolve
, and CanLoad
.
The utility of each of these guards plays a specific role in deciding the feasibility of routing and data retrieval.
Preloading Strategy
Apart from the application load, Angular offers certain strategies that can be used to preload certain modules in the background.
It optimizes the usability of the user interface enhancing the response time, yet the loading time is only minimally affected.
Angular also has different options for preloading, such as the An example of how to preload all modulesPreloadAllModules
option. import { PreloadAllModules } from '@angular/router';
RouterModule.forRoot(
[...],
{
preloadingStrategy: PreloadAllModules
}
)
Using this module you can create your preloading strategies to determine which modules should be preloaded. Among the optimizations, preloading of critical or the most often used modules improves application performance, particularly when shifting between the sections.
Through these practices, Angular application developers can gain better control over the performance of their application and make it scalable.
Best Practices
Here are the top angular best practices one should keep in mind while developing an angular application.
- Modular Design: Design the application that would allow them to account for future changes. Eliminate the possibility of mutual relationships between the features. Everyone should contain all its data and logic, meaning that it is easier to maintain and scale than if all the data and logic were mixed up.
- Eager Loading Critical Modules: The use of lazy loading assists in increasing performance but in some instances, certain modules such as security should at least be eagerly loaded to be available from the onset of the application. This means that basic functionalities are tested and are available for the user to use to immediately shoot the usability factor.
- Monitor Performance: Use the Angular performance monitoring capabilities to quantify the effects of a change. This is crucial in enhancing performance since the regular check can enable one to locate areas where there are constraints and enhance the workability of the software. Applications like Angular DevTools can help identify what areas of the application could use enhancement.
Troubleshooting Common Issues
Lazy loading, in turn, can produce some problems too. Every time you implement lazy loading, some issues may occur which are solvable with a little intelligence.
Here are a few tips to overcome the same:
Route Not Found
Make sure that all the paths in your route configuration correspond to the directory structure.
This minimizes the occurrence of ‘Route Not Found’ errors cause they critically affect the functionality of any site.
To avoid such instances, make sure that the routing configuration of the files and the directories of the files to be routed match fully.
Module Loading Errors
Check whether the syntax for the presence of loadChildren
is correct and whether the path to the modules is correct. This leads to module loading errors that can cause a lot of problems.
This is often why a lot of care needs to be taken during the verification of the paths and syntax of the modules that are to be lazy-loaded.
Performance Bottlenecks
Refer to browser tools, such as Angular DevTools, to find out the areas where most of its time is consumed by the main thread.
These analyses enable reductionist approaches to be made regarding lazy loading.
It also explains ways that can be adopted to manage these applications for maximum efficiency and optimal implementation of the best forms of lazy loading.
Advanced Techniques for Optimizing Lazy Loading
For more performance optimization of your Angular application, you can try preloading and/or dynamic imports for even more efficient lazy-loading.
Below is a list of some of the favorite techniques used to maximize the results from lazy loading applications.
Preloading
Preloading is where certain modules must be in the background after the application has loaded for the first time.
Angular has multiple preloading strategies which include the ‘PreloadAllModules’ as well as the custom strategies for preloading. This technique enhances the user experience, especially during subsequent navigation, by providing a fast impression with reduced load times.
For instance, to get preloading set up in the application, specify the RouterModule in the app-routing.module.ts
file:
import { PreloadAllModules, RouterModule, Routes } from '@angular/router';
const routes: Routes = [
{
path: 'admin',
loadChildren: () => import('./admin/admin.module').then(m => m.AdminModule)
}
];
@NgModule({
imports: [RouterModule.forRoot(routes, { preloadingStrategy: PreloadAllModules })],
exports: [RouterModule]
})
export class AppRoutingModule {}
Dynamic Imports
The dynamic module allows you to load a JavaScript module on the go using the ‘import()’ function.
This technique is beneficial for preloading large third-party libraries or rarely used modules.
import('./path/to/big-lib').then(lib => {
// Use the imported lib
});
Dynamically imported modules mean that you can start with a small bundle and load a large piece of code when necessary.
Approach Comparision
While the decision of picking which of the lazy loading is pending, consider the following factors:
- Application Size: In smaller applications, one simply requires standard lazy loading. This means that the modules are only loaded when they will be of use. However, larger applications can use such techniques as preloading where some important modules are initiated in the background to boost performance and interactivity.
- User Experience: Preloading modules enhance the interactive application user experience by decreasing wait time when reaching averagely popular parts of the app. This way the users can witness smoother transitions and faster response times. This thereby improves the overall usability of the system and the users’ satisfaction levels.
- Code Complexity: Dynamic imports allow for easier updates to new modules; however, changes to existing applications can be complicated and may slow down the application load time.
Here, the author must decide if the additional complexity and possible TH performance price are warranted with dynamic import in a given application.
Future Development
The Angular development team improves the overall performance and UI of an app through continuous updates in lazy loading with every new version.
These should mainly involve enhancements in the working mechanics of lazy loading, for instance, enhanced manner of handling dependent modules, efficient ways of loading modules, and compatibility with modern features of JavaScript.
This will allow the developer always to check the Angular roadmap to know the next features to expect or even check on the improvement of the lazy loading feature.
Lazy loading in Angular is safe in the hands of the Angular team that continuously works on enhancing the effectiveness of lazy loading version after version. This enables applicative developers to engage in refining the performance, interactivity, and design of their applications with the Further enhancement of this feature.
Conclusion
Lazy loading in Angular loads only necessary resources only when a particular page is being loaded. This technique also minimizes the number of requests in the initial loading stage thereby increasing response time.
Use loadChildren
in routing to load modules on the go after users click something, it also helps to group modules into directories to enhance scalability and maintainability.
Route guards including ‘CanLoad’ and ‘CanActivate’ manage the accessibility to modules depending on the permission while keeping the data safe.
Make use of lazy loading and see the enhancements of its performance and usability in Angular applications.